SQL Cheat Sheet - 9 Must-Known SQL Syntax for Beginners
Published on
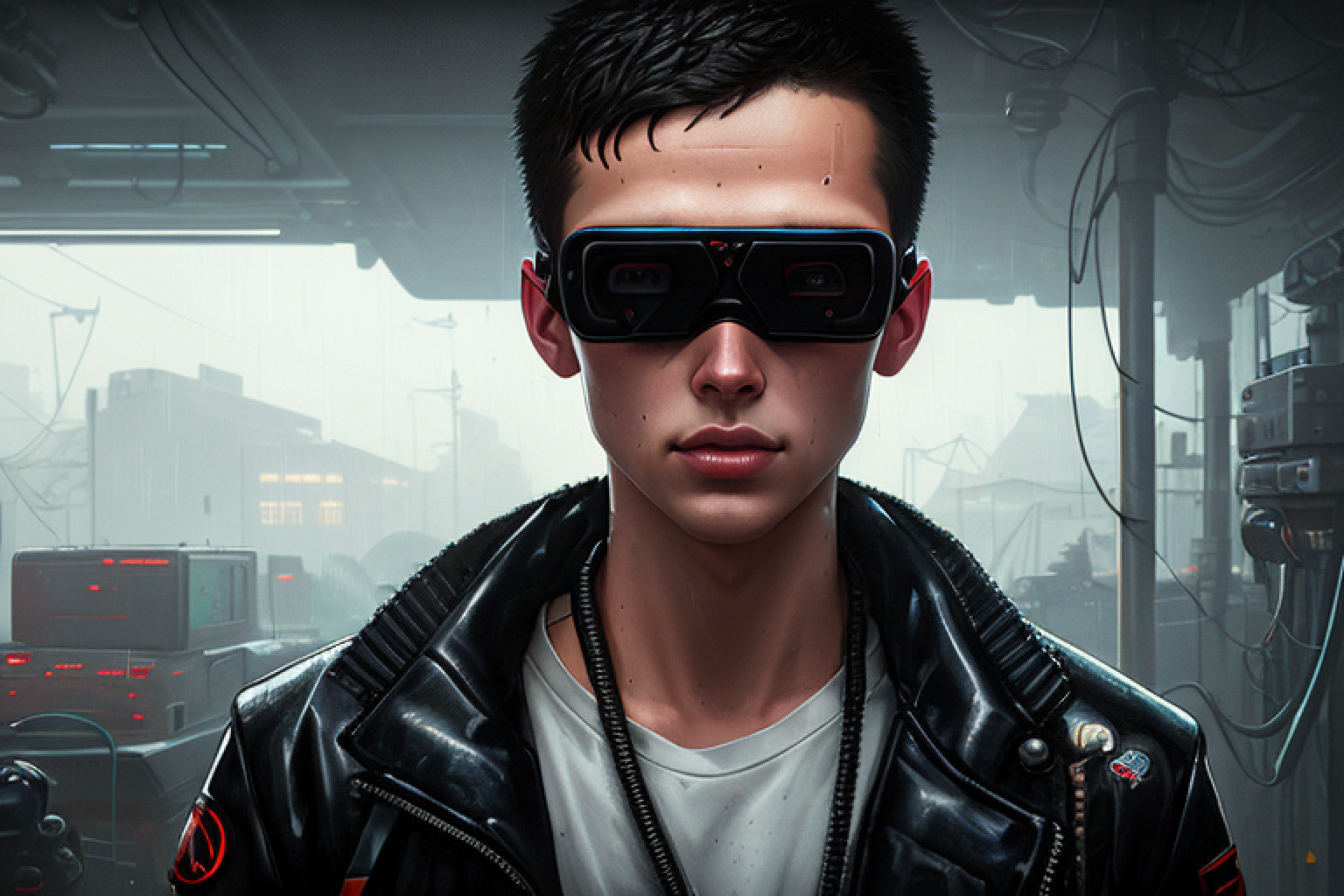
SQL (Structured Query Language) is a standard language used to manage relational databases. It is the most common language used for database management, and it is an essential skill for anyone working with databases. Whether you are a beginner or an experienced database developer, it is always useful to have a SQL cheat sheet to refer to when working with databases. In this article, we will provide you with a comprehensive SQL cheat sheet that covers the 10 most essential SQL syntax that every beginner should know.
9 Must-Known SQL Syntax for Beginners
1. SELECT Statement
The SELECT statement is the most commonly used SQL command and it is used to retrieve data from a database. It is the fundamental command used to retrieve data from a database. The syntax of the SELECT statement is as follows:
SELECT column1, column2, ...
FROM table_name;
Here, column1
, `column2, ... specify the columns you want to retrieve, and table_name specifies the table from which you want to retrieve the data.
2. WHERE Clause
The WHERE clause is used to filter the data returned by the SELECT statement. It allows you to specify conditions that must be met for the data to be returned. The syntax of the WHERE clause is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE column = value;
Here, column = value
specifies the condition that must be met for the data to be returned.
3. AND and OR Operators
The AND and OR operators are used in the WHERE clause to combine multiple conditions. The AND operator returns only the rows that meet all the conditions, while the OR operator returns the rows that meet at least one of the conditions. The syntax of the AND and OR operators is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE column1 = value1 AND column2 = value2;
SELECT column1, column2, ...
FROM table_name
WHERE column1 = value1 OR column2 = value2;
4. GROUP BY Clause
The GROUP BY clause is used to group the data returned by the SELECT statement based on one or more columns. It is often used with aggregate functions such as COUNT, SUM, AVG, MIN, and MAX. The syntax of the GROUP BY clause is as follows:
SELECT column1, COUNT(column2)
FROM table_name
GROUP BY column1;
Here, column1 is used to group the data, and COUNT(column2) returns the number of occurrences of column2 for each group.
5. HAVING Clause
The HAVING clause is used to filter the data returned by the GROUP BY clause. It allows you to specify conditions that must be met for the data to be returned. The syntax of the HAVING clause is as follows:
SELECT column1, COUNT(column2)
FROM table_name
GROUP BY column1
HAVING COUNT(column2) > value;
Here, COUNT(column2) > value specifies the condition that must be met for the data to be returned.
6. ORDER BY Clause
The ORDER BY clause is used to sort the result-set in ascending or descending order. This clause is optional and if you do not specify an ORDER BY clause, the result-set will be sorted in ascending order by default. The syntax of the ORDER BY clause is as follows:
SELECT column1, column2, ...
FROM table_name
ORDER BY column1 ASC|DESC, column2 ASC|DESC, ...;
In the above syntax, column1, column2, etc. are the names of the columns in the result-set, and ASC and DESC are used to specify the sorting order. If you use ASC, the result-set will be sorted in ascending order, and if you use DESC, the result-set will be sorted in descending order.
For example, let's say you have a table named students with the following data:
+----+--------+--------+
| id | name | marks |
+----+--------+--------+
| 1 | Alice | 90 |
| 2 | Bob | 80 |
| 3 | Charlie| 95 |
| 4 | Dave | 85 |
+----+--------+--------+
If you want to sort the result-set in ascending order by the marks column, you can use the following SQL statement:
SELECT *
FROM students
ORDER BY marks ASC;
The result-set will be as follows:
+----+--------+--------+
| id | name | marks |
+----+--------+--------+
| 2 | Bob | 80 |
| 4 | Dave | 85 |
| 1 | Alice | 90 |
| 3 | Charlie| 95 |
+----+--------+--------+
If you want to sort the result-set in descending order by the marks column, you can use the following SQL statement:
SELECT *
FROM students
ORDER BY marks DESC;
The result-set will be as follows:
+----+--------+--------+
| id | name | marks |
+----+--------+--------+
| 3 | Charlie| 95 |
| 1 | Alice | 90 |
| 4 | Dave | 85 |
| 2 | Bob | 80 |
+----+--------+--------+
In the above example, the result-set is sorted in descending order by the marks column, so the highest marks appear first.
It is important to note that the ORDER BY clause can sort the result-set based on multiple columns. For example, if you want to sort the result-set in descending order by the marks column, and then in ascending order by the name column, you can use the following SQL statement:
SELECT *
FROM students
ORDER BY marks DESC, name ASC;
The result-set will be as follows:
+----+--------+--------+
| id | name | marks |
+----+--------+--------+
| 3 | Charlie| 95 |
| 1 | Alice | 90 |
| 4 | Dave | 85 |
| 2 | Bob | 80 |
+----+--------+--------+
7. GROUP BY Clause
The GROUP BY clause is used to group the rows in the result-set based on the values in one or more columns. This clause is often used in combination with aggregate functions such as SUM, AVG, MIN, MAX, etc. to perform calculations on the grouped data. The syntax of the GROUP BY clause is as follows:
SELECT column1, aggregate_function(column2), ...
FROM table_name
GROUP BY column1;
In the above syntax, column1 is the name of the column based on which the rows are grouped, aggregate_function is an aggregate function such as SUM, AVG, MIN, MAX, etc., and column2 is the name of the column for which the aggregate function is performed.
For example, let's say you have a table named sales with the following data:
+----+--------+-------+
| id | date | sales |
+----+--------+-------+
| 1 | 2021-01-01 | 100 |
| 2 | 2021-01-02 | 150 |
| 3 | 2021-01-03 | 200 |
| 4 | 2021-02-01 | 175 |
| 5 | 2021-02-02 | 225 |
+----+--------+-------+
If you want to find the total sales for each month, you can use the following SQL statement:
SELECT DATE_FORMAT(date, '%Y-%m') AS month, SUM(sales) AS total_sales
FROM sales
GROUP BY month;
8. HAVING Clause
The HAVING clause is used to filter the result-set based on the conditions specified in the aggregate functions. It works similar to the WHERE clause but is used in conjunction with the GROUP BY clause. The syntax of the HAVING clause is as follows:
SELECT column1, aggregate_function(column2), ...
FROM table_name
GROUP BY column1
HAVING condition;
In the above syntax, column1 and aggregate_function(column2) are the columns and aggregate functions used in the SELECT statement, and condition is the condition to be met by the result-set.
For example, let's say you have a table named sales with the following data:
+----+--------+-------+
| id | date | sales |
+----+--------+-------+
| 1 | 2021-01-01 | 100 |
| 2 | 2021-01-02 | 150 |
| 3 | 2021-01-03 | 200 |
| 4 | 2021-02-01 | 175 |
| 5 | 2021-02-02 | 225 |
+----+--------+-------+
If you want to find the total sales for each month where the total sales is greater than 200, you can use the following SQL statement:
SELECT DATE_FORMAT(date, '%Y-%m') AS month, SUM(sales) AS total_sales
FROM sales
GROUP BY month
HAVING SUM(sales) > 200;
The result-set will be as follows:
+-------+-----------+
| month | total_sales |
+-------+-----------+
| 2021-02 | 400 |
+-------+-----------+
As you can see, only the month with total sales greater than 200 is returned in the result-set.
9. UNION Clause
The UNION clause is used to combine the result-sets of two or more SELECT statements into a single result-set. The syntax of the UNION clause is as follows:
In the above syntax, column1, column2, ... are the columns to be selected, and table_name1 and table_name2 are the names of the tables from which the data is to be selected.
For example, let's say you have two tables named sales1 and sales2 with the following data:
Table: sales1
+----+--------+-------+
| id | date | sales |
+----+--------+-------+
| 1 | 2021-01-01 | 100 |
| 2 | 2021-01-02 | 150 |
+----+--------+-------+
Table: sales2
+----+--------+-------+
| id | date | sales |
+----+--------+-------+
| 3 | 2021-01-03 | 200 |
| 4 | 2021-02-01 | 175 |
+----+--------+-------+
If you want to combine the data from both tables into a single result-set, you can use the following SQL statement:
SELECT date, sales
FROM sales1
UNION
SELECT date, sales
FROM sales2;
The result-set will be as follows:
+--------+-------+
| date | sales |
+--------+-------+
| 2021-01-01 | 100 |
| 2021-01-02 | 150 |
| 2021-01-03 | 200 |
| 2021-02-01 | 175 |
| 2021-02-02 | 225 |
+--------+-------+
As you can see from the result-set, the UNION clause has combined the data from both tables into a single result-set and eliminated any duplicate rows.
Conclusion
SQL is a powerful language for manipulating and retrieving data from databases. The SELECT statement is the most commonly used SQL statement, and the syntax and clauses covered in this cheat sheet will help you get started with writing SQL queries. Whether you're a beginner or an experienced SQL user, this cheat sheet is an excellent reference for the most commonly used SQL syntax.